Setting Up Environment Variables
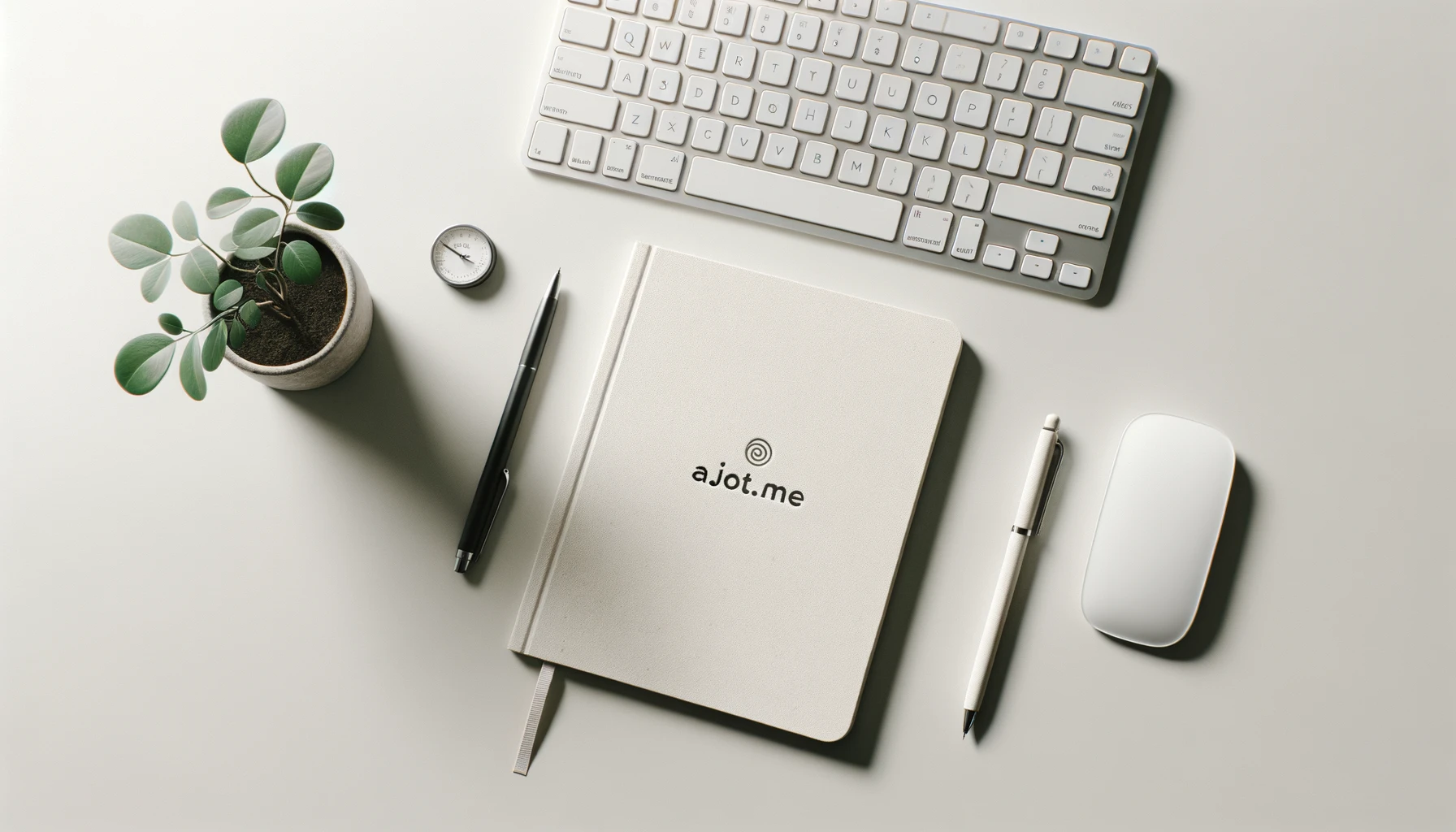
Setting Up Environment Variables to store API Keys Safely in Python Virtual Environments
Introduction
Working with APIs often involves handling sensitive keys. Hardcoding them in your main.py
is not only risky but also a potential privacy nightmare, particularly if you accidentally push your code to public repositories like GitHub.A safer, more professional approach is using environment variables.
Let’s break down how to do this within a Python virtual environment.
Two Ways to Set Up Environment Variables
When it comes to setting up environment variables for your Python projects, there are two main approaches: globally across your system or locally within each project.
Understanding the Risks of Global Environment Variables
Setting your API keys globally means they’re accessible across all your projects on your machine. On the surface, this seems like a convenient option, eliminating the need to set up keys for each individual project.
However, this is not the recommended approach. Here’s why -
- Conflict Between Projects: Global keys can lead to conflicts, particularly in scenarios where different projects require unique API keys.
- Security Concerns: There’s a significant security risk with global variables. If one key is compromised, it can potentially jeopardize all your projects.
A more secure and organized method is to manage your API keys similarly to how you handle project-specific libraries, i.e., within virtual environments. By doing so, you ensure that each project maintains its own set of keys, fostering a more secure and orderly development environment.
Step 1: Setting Up a Virtual Environment
Create a virtual environment for your project. It’s a simple command:
python3 -m venv myenv
This command creates an isolated environment, myenv
, for your project.
Step 2: Adding API Keys to the Virtual Environment
Once your environment is ready, activate it:
source myenv/bin/activate
Now, set your API key:
export MY_API_KEY='your-api-key'
[Optional] Automatically Making API Key Available in Virtual Environments
To simplify your workflow, you can also add the line export MY_API_KEY='your-api-key'
directly to the myenv/bin/activate
script.
This ensures that your API key is automatically set and ready each time you activate your virtual environment.
[Optional] Test if the environment variable is working
Once you have set the MY_API_KEY environment variable in your terminal, you can quickly test to ensure it’s working correctly.
In the terminal, type echo $MY_API_KEY
If the terminal does not display anything, it means the environment variable is not set in the current terminal session.
Step 3: Using the API Keys in Your Application
In your Python code, access the API key like this:
import os
api_key = os.getenv('MY_API_KEY')
I hope this guide proves helpful. Happy coding!